Test automation: What it is and how to implement
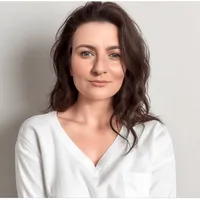
Taylor Bruneaux
Analyst
Verifying a simple code’s accuracy, reliability, and scalability can prevent you from shipping your product quickly and efficiently. This challenge grows in scale along with our systems. How can you verify a new release quickly and with minimal cost before shipping it live to production?
One key weapon in your arsenal is test automation. In this article, we’ll examine test automation, the different types of automation available, and the benefits and challenges of implementing it in practice.
What is test automation?
Test automation is a software verification technique in which you run code to validate the functionality of your system every time someone checks in a new change. Based on the results of these tests, either your test code or a human operator decides whether to deploy this change to the next stage.
Test automation is part of a continuous integration and continuous deployment (CI/CD) build system, which automates running tests and pushing code through various deployment stages as part of a gradual rollout to production.
A software system’s test suite is the total of its tests. Teams capture and evaluate statistics on their test suites - e.g., percentage of passed vs. failed tests, time taken to run the test suite, performance of an individual test case, etc. - to evaluate a system’s overall stability and performance.
A typical automation testing workflow might look like this:
- A developer writes tests to verify code changes made to a system, such as adding a new feature.
- After verifying a code change on their local environment, a developer checks in their change to source control and creates a pull request.
- As the developer waits to review their pull request, the CI/CD system runs the new and all previous tests to verify the change. If there are any failures, the developer creates and checks in fixes to the PR.
- After a reviewer approves the pull request, the CI/CD system deploys the change to a test environment.
- The CI/CD system assesses the test environment to verify that the change works as expected. If the tests fail, the system rolls back the changes. If they pass, the system deploys the change to the next stage (e.g., pre-production, production).
Continuous testing
Continuous testing is an approach to software testing that emphasizes automated testing integration throughout the software development lifecycle. Unlike traditional testing methods, which occur in isolated phases, continuous testing advocates for test coverage early, often, and consistently across all stages of development, from code commit to production deployment. By automating test execution and analysis within continuous integration and continuous delivery (CI/CD) pipelines, continuous testing enables rapid feedback on code changes, detects defects early, and ensures that software meets quality standards at every iteration. This iterative and proactive test coverage approach helps mitigate risks, accelerate release cycles, and deliver high-quality software products that meet user expectations.
Types of test automation
A test automation framework can automate various tests at different development, integration, and deployment points. These include:
Unit testing
Unit tests test a specific code path, such as a function or method. The goal of a unit test is to test just that code in isolation, independent of any dependencies.
When developers work on a code path that relies on external dependencies, such as data retrieval from a database or an API call, they usually create a mock or stub interface for these dependencies. These interfaces help avoid confusing errors in these dependencies and the newly written code.
A CI/CD pipeline will typically run as a test case any time a developer checks in new code.
Integration testing
As its name implies, integration tests validate a change in the context of the entire system, with all of its internal and external dependencies. An example would be calling an API endpoint in a test or staging environment.
Whereas unit tests validate a single code path, integration tests validate that a change works well with your system’s other moving parts.
Smoke testing
Running a full test suite can be time-consuming - and expensive. Teams can waste a lot of time and money running a test suite for an hour only to have the suite fail on a basic test.
To prevent this, some teams develop smoke tests that validate a system’s basic functionality before commencing further automation testing. The name comes from the hardware world, where engineers would turn a device on to ensure it didn’t spew smoke.
Performance testing
Performance testing is crucial for determining a system’s efficiency. It helps us understand how fast the system responds, how much work it can handle, and how it can adapt to different scenarios.
There are different kinds of performance tests, such as stress testing, which looks at how the system works when it’s overloaded, and load testing, which checks if the system can handle the expected workload. Endurance testing is another type, which examines how long the system can keep up its performance without slowing down. We might also use other specialized performance tests to make sure the system performs well in all real-world situations.
Security testing
Security testing ensures that software meets all defined standards for authorized access and does not expose known flaws. Security testing is a large umbrella area that we can break into two major categories:
- SAST, or static application security testing, analyzes source code, binaries, and other assets for known vulnerabilities. (Examples: Credentials included in source code; older versions of application binaries with known security risks)
- DAST, or dynamic application security testing, exploits security vulnerabilities in running applications. (Example: A REST API endpoint that doesn’t enforce authorization)
SAST runs during the application build process. After deployment to a specific stage, DAST runs on the application.
Regression testing
Regression testing attempts to ensure that new changes do not re-introduce known bugs. It’s less a type of automation testing and more of a policy that any previously found defects should be encoded as tests and run with every build or deployment. Regression testing is essential as it prevents you from learning the same expensive lesson twice.
Benefits of test automation
Increases release quality
As you add more performance tests to your test suite, it becomes more comprehensive and covers more critical elements of your system. These additions increase the quality of your releases by weeding out expected and known bugs early in the software development lifecycle.
Increases release cadence
Test automation reduces the amount of human time devoted to test management. It also enables you to reduce linear testing time through techniques such as parallelization (i.e., running two distinct parts of your test suite simultaneously on different computing instances).
Automation testing can also reduce the time required to deploy a new release by finding defects earlier in the software development lifecycle when they’re easier to fix and re-deploy than if you had found the defect in production.
Reduces bug fix cost
Studies (such as this by Rudolf van Megen & Dirk B. Meyerhoff) show that early defect detection reduces the injection of significant defects into a system and makes fixing those that remain less expensive.
Decreases technical debt
Technical debt represents the overhead required to make changes to a system due to shortcuts or compromises taken in the past. Studies like “The Influence of Technical Debt on Software Developer Morale” have found a correlation between technical debt and decreased morale and developer productivity.
Unit testing can help decrease technical debt, making it easier and faster to implement changes to a system. That’s because unit-tested code tends to be better designed and more modular.
Challenges with test automation
While test automation has multiple benefits, implementing and maintaining a test automation suite has unique struggles.
Making testing a priority
Testing is one of those things, like technical documentation, that teams tend to cut when a project runs late. It takes time to prioritize automation testing and accounting for its results in project planning and delivery estimates.
Test suite bloat
Some teams end up with the opposite problem. They get into automation testing and end up with a large, hard-to-maintain test suite that takes an hour or more to run. This bloat can lead to slower releases due to long build times, overwhelming test data, and the need to keep tests updated as the code base evolves.
Flaky tests
A flaky test can pass or fail even when the underlying code hasn’t changed. When failures occur, it is often due to inadequate setup teardown of tests or temporary network issues that the code cannot handle effectively. For instance, it may not implement retries for a failed HTTP call.
Flaky tests can have several negative consequences. The key downside is that they make your CI/CD system less effective, lowering productivity and reducing test efficiency. They also encourage “test failure blindness,” a phenomenon where developers ignore failed tests because they don’t believe the test suite is reliable. If you don’t deal with flaky tests, they can eventually harm your entire automation testing effort.
How to get started with test automation
Test automation isn’t all or nothing. If you don’t already have a test automation strategy, you can define and evolve one over time by following these steps.
Define your goals
Talk with your team about what you want from automation testing. Common goals include reducing time spent on manual testing, improving software quality, accelerating release cycles, and reducing the mean cost per defect.
Decide on essential tests for automation
Tests that are time-consuming, repetitive, and prone to human error are usually the best candidates for automation.
Select tools and frameworks.
Choose appropriate tools and frameworks based on your application’s technology stack and your team’s skillset. Consider language support, community support, licensing costs, and integration capabilities.
Set up your test environment
If your CI/CD pipeline doesn’t already have a dedicated test environment, work on spinning one up using a new or existing Infrastructure as Code (IaC) template. This template will include setting up everything your test environment needs, including databases, networks, virtual machines, and any dependent services.
Your test environment should be separate from the dev and production environments to prevent adverse impacts on developers and users. If you template this environment using IaC, you can save money on automation testing by dynamically setting it up and tearing it down on demand as part of your CI/CD pipeline.
Start small
Don’t feel you have to boil the ocean. Start with one test case, then expand to a handful of your most critical tests.
Adopt best practices for automation testing
To maintain a consistent test environment, keep your tests independent, make them repeatable, and ensure they clean up after themselves. Use an automation testing tool with version control for your test scripts and integrate your automated tests with your CI/CD pipeline for continuous testing.
Scale your automated testing efforts
As you expand your test suite, focus on the use cases representing how users typically interact with your system, giving you the highest return on investment. Add regression tests for any issues found in production for which you had to deploy fixes. Remember to modify your tests as you evolve the underlying code base so that they stay relevant and stable.
Measure and evaluate
Implement a reporting mechanism to track the success and failure of your tests. Reporting can help you quickly identify issues and assess the quality of your application. Use dashboards or integrate with your project management tools to inform stakeholders about test outcomes.
To show the business value of test automation, measure how it impacts product quality over time. This can include measuring the number of production incidents, the mean time required to ship a change, the number of releases shipped, the mean time to failure, and the number of defects found at each deployment stage.
Evangelize and standardize your automated testing process and tools
After providing automated testing with one team, work on evangelizing it throughout the organization. Provide a standard set of tools that teams can learn quickly and drop into their own CI/CD pipelines to lower the barrier to onboarding.
Testing automation tools
Testing automation tools are crucial in streamlining the software testing process, enabling organizations to achieve higher efficiency, accuracy, and reliability in their testing endeavors. These tools encompass various functionalities, from test case management and execution to performance testing and security scanning. By automating repetitive tests and providing comprehensive reporting capabilities, these tools empower teams to deliver high-quality software faster.
Here are some popular test automation tools:
Selenium
One of the most widely-used open-source testing frameworks, Selenium facilitates automated testing of web applications across various browsers and platforms. This test automation tool offers a suite of tools for different testing needs, including Selenium WebDriver for browser automation and Selenium Grid for parallel testing. Selenium’s flexibility, robustness, and extensive community support make it a popular choice for web application testing.
Appium
Appium is an open-source automation tool designed for mobile application testing. It allows testers to automate native, hybrid, and mobile web apps on iOS and Android platforms. Appium leverages standard automation APIs and provides cross-platform compatibility, enabling seamless testing of mobile applications across different devices and operating systems.
JUnit
A widely used testing framework for Java applications, JUnit simplifies unit test execution. It provides annotations and assertions for defining test cases, along with features like parameterized tests and automated test suites for organizing and executing tests efficiently. JUnit’s integration with build tools like Maven and Gradle makes it essential for Java developers to practice test-driven development (TDD).
Postman
Postman is a collaborative platform primarily used for API testing that allows testers to design, automate, and document API requests. With features like automated test scripts, pre-request scripts, and assertions, Postman enables testers to automate the validation of API responses, performance testing, and integration testing. Its user-friendly interface and extensive collection of plugins make it a preferred choice for API test execution across industries.
JMeter
Apache JMeter is a versatile performance testing tool capable of simulating heavy loads on servers, networks, and objects to assess their performance under various conditions. JMeter supports testing web applications, databases, FTP servers, and more, offering features like distributed testing, dynamic parameterization, and result analysis. Its test data scalability, extensibility, and support for various protocols make it a valuable asset for performance engineers.
Common misconceptions about test automation
Despite its widespread adoption and proven benefits, test automation is often subject to misconceptions that can hinder its effective implementation and utilization. Addressing these misconceptions is essential for ensuring that organizations fully realize the potential of test automation in improving software quality and accelerating development cycles.
Test automation eliminates the need for manual testing.
There’s a common misunderstanding that automated testing can completely replace manual testing. While automated testing can make repetitive and time-consuming testing tasks more efficient, it can’t replace manual testing’s critical thinking, creativity, and exploratory nature.
Manual testing is still crucial for situations that require human judgment, such as usability testing, exploratory testing, and edge case analysis. A balanced approach combining manual and automated testing methods is necessary for comprehensive test coverage and adequate quality assurance.
Test automation guarantees flawless software.
Automated testing is sometimes thought to be a foolproof way to ensure that software is completely free of defects. However, this is not entirely accurate. Although automation can quickly identify certain bugs, it cannot always detect every possible defect or guarantee that the software is completely bug-free.
The effectiveness of automated testing is dependent on the test cases it executes, which must be designed carefully, with expert knowledge of the domain and continuous refinement. Automated testing may also miss subtle bugs or behavioral nuances that only manual testing can reveal. Therefore, while automated testing can help improve quality assurance efforts, it is not a substitute for rigorous software testing practices and ongoing improvements.
Test automation is too costly and time-consuming to implement
Some organizations are hesitant to use test automation due to its perceived costs and time constraints. However, while there may be initial expenses in selecting automation tools, training, and setting up infrastructure, the long-term benefits of functional testing far outweigh the upfront costs.
Automated tests can significantly reduce the time and effort required for regression testing, speed up release cycles, and lower the risk of post-deployment defects. Additionally, advances in automation frameworks, cloud-based testing platforms, and collaboration tools have made test automation more accessible and cost-effective for organizations of all sizes.
How test automation contributes to a great developer experience
Test automation isn’t just about testing; it’s about turbocharging your developer experience. Imagine a world where your code changes are instantly validated, where developers catch bugs before they even have a chance to blink. That’s the power of automated testing—it accelerates your software engineering feedback loop to warp speed. With an automated test running in the background, developers can iterate fearlessly, knowing that any missteps will be caught and corrected in real time.
But it’s not just about speed; it’s about reclaiming developers’ time and mental bandwidth. Gone are the days of manually executing tests for every code tweak. With automated testing handling the heavy lifting, developers can focus on what they do best – solving complex problems and pushing the boundaries of innovation.
And let’s not forget the impact on team dynamics. Automated testing is the ultimate documentation, ensuring everyone is on the same page about what constitutes a passing codebase. This clarity fosters smoother collaboration, fewer misunderstandings, and, ultimately, happier developers.