Enhance your code quality with our guide to code review checklists
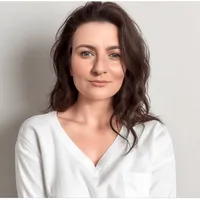
Taylor Bruneaux
Analyst
A robust code review process enhances code quality and team cohesion, making it a crucial step for all effective engineering teams to take before deployment. By focusing on the benefits of code reviews, teams can ensure that each piece of code meets high standards while improving collaboration.
This post explores what makes peer code review genuinely effective rather than time-consuming. We’ll examine practices for code review that go beyond superficial checks to address what truly matters in your source code, ensuring a smoother developer workflow.
We’ll discuss balancing thoroughness with keeping things moving and examine how senior engineers can use reviews as teaching moments without becoming bottlenecks. Attention to consistent naming conventions and avoiding anti-patterns can streamline feedback while providing additional context to help clarify complex changes.
We’ll cover practical performance considerations that reviewers should look for and how junior developers can grow through constructive feedback in a review process. By taking systematic review approaches, teams can build better code and better engineers.
What is a code review?
A code review is a structured process where developers evaluate new or modified code before merging it into the main codebase. Following code review guidelines helps ensure quality, maintainability, security, and performance while fostering collaboration and knowledge sharing. An effective review process not only catches bugs and prevents technical debt but also aligns the team with best practices and enhances the developer experience.
A good review starts with code quality. The code should be readable, maintainable, and adhere to established coding standards.
Reviewers should watch for unnecessary complexity, enforce modular design, and identify areas that might accumulate technical debt. Using static analysis tools can help catch issues early, while proper technical documentation ensures that future developers can understand and extend the code.
Security is a key part of code review. Developers should look for issues like SQL injection, XSS, and CSRF. They should check input sanitization, authentication, and sensitive data handling. Ignoring security can lead to serious risks in production.
Error handling and performance also deserve close attention. Code should gracefully handle failures, with meaningful error messages, proper logging, and well-structured exception handling.
Performance bottlenecks should be flagged by analyzing database queries, memory usage, and execution time, using profiling tools where necessary.
Code review best practices
A well-structured code review process improves code quality, security, and collaboration while preventing costly issues. Focus on a systematic approach to make code reviews efficient, consistent, and valuable.
Set clear goals and expectations
Before implementing a code review process, define what success looks like:
- Use a comprehensive code review checklist to ensure every code meets security, performance, and maintainability standards. We provide some jumping-off points for creating your own code review checklist below.
- Define review responsibilities—who should review what? Senior engineers should handle architectural decisions, while junior developers can review straightforward changes.
- Ensure security issues and performance considerations are always part of the review process, not afterthoughts.
Automate repetitive checks to speed up reviews
Code reviewers should focus on business logic, security risks, and architecture, not formatting issues or rote tasks. Automate with code review tools to reduce review time and prevent unnecessary context switching.
- Use static code analysis and review automation to catch format code issues, potential performance bottlenecks, and type hinting issues before humans review them.
- Enforce style guide rules automatically to avoid wasting time on stylistic feedback.
- All unit and integration tests must pass before a review begins.
- Scan dependencies for vulnerabilities to prevent security flaws from entering production code.
Keep pull requests small and reviews focused
Large pull requests slow down reviews, increase context switching, and make it harder to catch potential issues. Smaller, well-structured reviews lead to faster approvals, better feedback, and smoother code flow.
- Aim for pull requests under 400 lines of code to improve focus and review time efficiency.
- Encourage small, frequent reviews instead of significant and overwhelming changes.
- Limit review sessions to 60 minutes to maintain focus and prevent messy code review processes.
- Prioritize reviewing business logic and structural decisions before minor changes.
Make feedback constructive and actionable
A strong feedback culture helps developers work together smoothly and improves code quality by promoting best practices and reducing technical debt.
- Emphasize providing specific and actionable feedback instead of general comments. For instance, rather than saying, “This function is too complex,” you could say, “You might want to break this into smaller, independent functions to improve clarity.”
- Balance critical feedback with positive reinforcement to encourage continuous improvement.
- If feedback is subjective or relies on personal preferences, consider project conventions.
- Use pre-defined issue checklists, like those below, to avoid nitpicking and focus on essential issues.
Continuously improve the process
No peer review process is perfect—teams should continuously refine their approach to prevent inefficiencies.
- Experienced developers and code authors should regularly gather feedback on what works and what doesn’t.
- Track success metrics like review time, defect rates, and resource bottlenecks to identify inefficiencies.
- Update the code documentation and review the checklist based on past findings.
Example code review processes and checklists
While an essential checklist covering syntax correctness and coding standards is a great starting point, high-quality code reviews go beyond these fundamentals.
Effective reviews address:
- Modularity and reusability
- Integration testing
- Performance bottlenecks
- Robust error handling
- Security best practices
- Documentation evaluation
- Anti-pattern identification
Below are several structured checklists covering different aspects of a thorough code review.
General code review checklist
Use this checklist for any code review to ensure the code is maintainable, well-documented, and free from obvious errors.
Code quality & maintainability
- Adheres to project standards
- Follows modular programming principles
- Uses meaningful variables, functions, and class names for readability
- Technical documentation is clear and up-to-date
Error handling & logging
- Uses try-catch blocks appropriately
- Logs errors without exposing sensitive information
Testing & validation
- Unit tests cover core functionality and edge cases
- Automated tests are integrated into CI/CD pipelines
Security code review checklist
Use this checklist when reviewing the code base for security vulnerabilities, authentication, and data protection.
Input validation & injection prevention
- Validates all input for type, length, and format
- Protects against SQL Injection & XSS
Authentication & authorization
- Uses secure password storage (bcrypt, Argon2)
- Implements least privilege access principles
Sensitive data handling
- Encrypts sensitive data at rest and in transit
- Avoids logging or exposing sensitive data in URLs
Dependency management
- Ensures third-party libraries are updated and checked for vulnerabilities
Performance code review checklist
Use this checklist when evaluating code efficiency, resource usage, and scalability.
Algorithm & query efficiency
- Code is optimized for time and space complexity
- SQL queries are indexed and avoid N+1 problems
Memory & resource management
- Efficient memory allocation, preventing leaks
- Avoids excessive object retention and redundant processing
Concurrency & caching
- Uses threading & concurrency efficiently
- Implements caching for frequently accessed resources
API & integration code review checklist
Use this checklist when reviewing backend service API design, security, and performance.
API design & usage
- Follows RESTful or GraphQL best practices
- API responses use appropriate HTTP status codes
Security & authentication
- Uses OAuth, API keys, or JWT for authentication
- API requests are properly validated
Error handling & performance
- API provides meaningful error responses
- Minimizes and compresses payload sizes
Frontend code review checklist
Use this checklist when reviewing UI components, client-side performance, and accessibility.
Code structure & maintainability
- Uses a modular component structure
- Adheres to accessibility best practices (WCAG compliance)
Performance optimization
- Minimizes unnecessary re-renders and optimizes assets
- Implements efficient event handling
Security
- Frontend validation complements backend security
- No sensitive keys or credentials are exposed
Mobile app code review checklist
Use this checklist when reviewing mobile applications for UX consistency, battery efficiency, and offline handling.
UI/UX & performance
- Adheres to platform-specific design guidelines
- UI is responsive across different screen sizes
Memory & battery optimization
- Manages memory efficiently to avoid excessive battery drain
Offline handling
- Gracefully handles network disconnections
- Uses caching for improved performance
How the code review process improves developer productivity
A well-executed code review process improves developer productivity by enhancing speed, effectiveness, quality, and business impact—the four dimensions of the DX Core 4. It reduces defects early, promotes knowledge sharing, and ensures development teams build and ship software more efficiently. Implementing a code review process helps maintain a smooth code flow and reduces bottlenecks in the developer workflow.
Faster development without rework
Effective code reviews catch issues early, preventing costly rework. They improve deployment speed by ensuring code changes are high-quality the first time, reducing time spent debugging and refactoring later. Attention to naming conventions and modular code ensures clarity and maintainability.
Better knowledge sharing and team effectiveness
Reviews help developers learn from each other, spreading best practices and reducing reliance on individual expertise. Junior engineers ramp up faster, and senior developers reinforce design patterns and architectural principles across the team. Discussions on problematic language idioms and language features lead to better coding habits.
Higher code quality and fewer defects
Well-structured reviews enforce consistency and prevent security, performance, and reliability issues. They minimize post-release incidents, reduce firefighting, and allow developers to focus on new work. Code reviews ensure consistent coding styles, reducing confusion across the entire team.
Greater business impact through collaboration
A strong code review culture leads to better engineering decisions and alignment with business goals. Teams that catch issues early and build maintainable software create more reliable products with fewer disruptions. Addressing minor issues early in the process fosters a positive culture where developers provide valuable feedback, improve collective code ownership, and ensure efficient code reviews that focus on both quality and maintainability.
Code reviews go beyond finding bugs; they help build a stronger, faster, and more effective engineering team while ensuring a high-quality bar for software development.